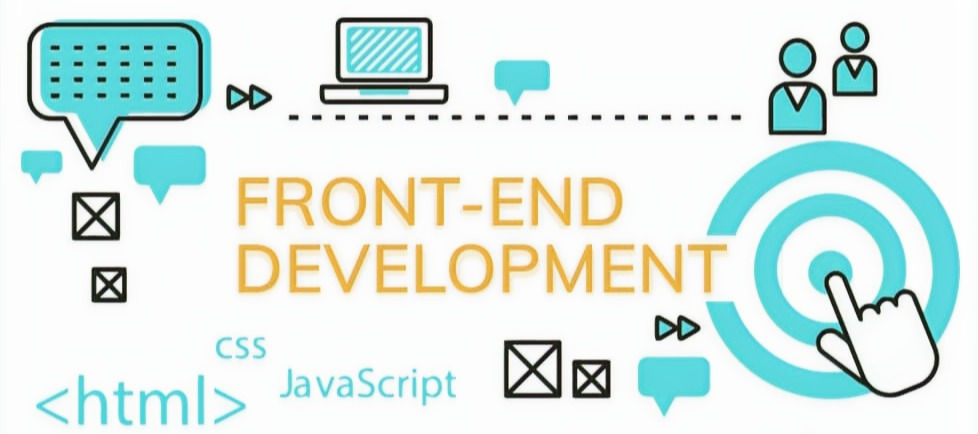
Front-end development is a pivotal aspect of web development, focusing on crafting the graphical user interface (GUI) and interactive components of websites. This field is crucial for translating complex data into a visually appealing and user-friendly format. Key technologies in front-end development include HTML, CSS, and JavaScript. HTML structures the content, CSS enhances the presentation with styles, and JavaScript adds interactivity and dynamic behavior.
In this article, we will explore the significance of front-end development in creating engaging web experiences. We will delve into the roles of HTML, CSS, and JavaScript, illustrating their individual and combined contributions to building robust web pages. Additionally, we will discuss advanced frameworks and responsive design principles, essential for optimizing websites across various devices and screen sizes. Through practical examples and code snippets, we aim to provide a comprehensive understanding of front-end development and its integral role in modern web development.
Understanding Front-End Development
Front-end development is the cornerstone of website development, responsible for crafting the user experience by converting data into a visually engaging and interactive format. It involves creating the graphical user interface (GUI) that users interact with directly. The three fundamental technologies that underpin front-end development are HTML, CSS, and JavaScript.
HTML (HyperText Markup Language) structures the web content, defining elements such as headings, paragraphs, and links. For instance, a simple HTML snippet might look like this:
<!DOCTYPE html>
<html>
<head>
<title>Sample Page</title>
</head>
<body>
<h1>Welcome to My Website</h1>
<p>This is a sample paragraph.</p>
</body>
</html>
CSS (Cascading Style Sheets) enhances the presentation by controlling layout, color, and typography. For example:
body {
font-family: Arial, sans-serif;
margin: 0;
padding: 0;
}
h1 {
color: navy;
}
p {
color: gray;
}
JavaScript adds interactivity and dynamic behavior to web pages. Here’s a simple example:
document.querySelector('h1').addEventListener('click', function() {
alert('You clicked the heading!');
});
Responsive design and mobile optimization are vital considerations in front-end development, ensuring that websites function seamlessly across various devices and screen sizes. Techniques like media queries in CSS and flexible grid layouts are employed to achieve responsiveness, enhancing user accessibility and experience.
Front-end development integrates HTML, CSS, and JavaScript to build visually appealing and interactive web pages, with an emphasis on responsive design to cater to the diverse landscape of modern devices.
The Building Blocks of Front-End Development
In front-end development, buttons, links, and animations are essential components that significantly enhance user experience and engagement. These elements not only contribute to the aesthetics of a website but also play crucial roles in its functionality and interactivity.
Buttons are vital for user interactions, serving as triggers for actions such as form submissions and navigation. Creating user-friendly buttons involves utilizing HTML for structure and CSS for styling. For example:
HTML:
<button class="btn-primary">Submit</button>
CSS:
.btn-primary {
background-color: #007bff;
color: white;
border: none;
padding: 10px 20px;
cursor: pointer;
}
Links facilitate navigation, guiding users through different sections of a website. Properly styled links enhance usability. An example of a styled link:
HTML:
<a href="https://www.example.com" class="nav-link">Home</a>
CSS:
.nav-link {
color: #007bff;
text-decoration: none;
}
.nav-link:hover {
text-decoration: underline;
}
Animations add a dynamic layer to web design, capturing user attention and making interactions more engaging. CSS animations can create smooth transitions, as shown below:
CSS:
@keyframes fadeIn {
from { opacity: 0; }
to { opacity: 1; }
}
.animated-element {
animation: fadeIn 2s ease-in-out;
}
Incorporating these elements effectively requires a blend of technical skill and creative design. By mastering buttons, links, and animations, front-end developers can create intuitive, visually appealing, and highly interactive web interfaces.
Tools and Technologies in Front-End Development
In front-end development, a variety of tools and technologies are essential for creating robust and dynamic web interfaces. Content management systems (CMS) like WordPress, Joomla, and Drupal play a significant role by providing frameworks that streamline the development process.
WordPress is a widely-used CMS known for its user-friendly interface and extensive plugin ecosystem. It enables developers to create custom themes and plugins, enhancing the functionality and aesthetics of websites. Joomla offers more flexibility and complexity, suitable for more advanced sites requiring extensive customization. Drupal, known for its powerful taxonomy and scalability, is ideal for large-scale websites with complex data structures.
Beyond CMS, front-end frameworks such as React, Angular, and Vue.js have revolutionized front-end development. React, developed by Facebook, allows developers to create reusable UI components, facilitating efficient and maintainable code. Angular, by Google, is a comprehensive framework that provides robust tools for building single-page applications (SPAs). Vue.js, known for its simplicity and flexibility, offers a progressive framework that can be integrated incrementally into projects.
javascript:
// Example of a simple React component
import React from 'react';
function Greeting() {
return <h1>Hello, World!</h1>;
}
export default Greeting;
Version control systems, such as Git, are crucial for collaborative front-end projects. They enable multiple developers to work simultaneously on different parts of a project, track changes, and merge code efficiently. GitHub and GitLab are popular platforms that provide version control and additional features like issue tracking and continuous integration.
Incorporating these tools and technologies into front-end development workflows enhances productivity and code quality. CMS platforms like WordPress, Joomla, and Drupal simplify content management, while frameworks like React, Angular, and Vue.js streamline the development of interactive and responsive user interfaces. Version control systems ensure efficient collaboration, making them indispensable for modern front-end development projects.
The Role of HTML, CSS, and JavaScript in Front-End Development
In front-end development, HTML, CSS, and JavaScript form the foundational triad that powers web interfaces. Each technology plays a distinct and critical role in creating functional and visually appealing websites.
HTML5: The Backbone of Web Content
HTML5 is the latest iteration of the HyperText Markup Language, designed to structure web content. Its evolving standards introduce a range of new elements and attributes that enhance semantic meaning and accessibility. For example, <article>, <section>, and <nav> tags offer more meaningful structure compared to generic <div> tags, thus improving SEO and accessibility. Clean, semantic HTML code ensures that search engines can better understand and index content, leading to improved visibility in search results.
<article>
<header>
<h1>Understanding HTML5</h1>
<p>By John Doe</p>
</header>
<section>
<h2>Introduction</h2>
<p>HTML5 introduces new elements...</p>
</section>
</article>
CSS3: Crafting the Visual Experience
CSS3 is the latest version of Cascading Style Sheets, responsible for the presentation layer of web pages. Its advanced features, such as Flexbox, Grid, and animations, provide developers with powerful tools to create responsive and visually engaging designs. CSS3 also supports media queries, enabling the creation of responsive designs that adapt seamlessly to various screen sizes.
Example of a responsive layout using CSS Grid:
.container {
display: grid;
grid-template-columns: repeat(auto-fit, minmax(200px, 1fr));
gap: 20px;
}
JavaScript: Bringing Interactivity to Life
JavaScript is the scripting language that adds interactivity and dynamic behavior to web pages. It allows developers to create responsive interfaces, handle events, and manipulate the DOM (Document Object Model). Modern JavaScript frameworks and libraries, such as React, Angular, and Vue.js, further enhance the capabilities of front-end development by providing robust tools for building complex user interfaces.
Example of a simple JavaScript function:
document.getElementById("button").addEventListener("click", function() {
alert("Button clicked!");
});
Ensuring Accessibility and Optimization in Front-End Development
In the competitive landscape of front-end development, ensuring accessibility and optimizing performance are crucial for delivering high-quality web experiences. These practices not only enhance user satisfaction but also improve search engine rankings and overall website efficiency.
Best Practices for Optimizing Front-End Code
Optimizing front-end code is vital for improving website performance and loading speed. Here are some key strategies:
Minify and Compress Files
Minifying HTML, CSS, and JavaScript files reduces file size by removing unnecessary whitespace, comments, and code. Tools like UglifyJS for JavaScript and CSSNano for CSS are commonly used.
Example of CSS minification:
/* Original CSS */
body {
margin: 0;
padding: 0;
}
/* Minified CSS */
body{margin:0;padding:0;}
Use Asynchronous Loading for JavaScript
Loading JavaScript files asynchronously prevents them from blocking the rendering of the web page. This can be achieved by adding the async attribute to script tags.
Example:
<script src="script.js" async></script>
Optimize Images
Large images can significantly slow down a website. Use modern formats like WebP and ensure images are appropriately sized and compressed. Tools like ImageOptim or TinyPNG can be helpful.
Example of optimized image usage:
<img src="image.webp" alt="Descriptive Alt Text" width="600" height="400">
Implement Lazy Loading
Lazy loading defers the loading of non-essential resources until they are needed. This is particularly useful for images and iframes.
Example:
<img src="image.jpg" loading="lazy" alt="Lazy Loaded Image">
Enhancing Accessibility
Accessibility in front-end development ensures that web content is usable by all, including individuals with disabilities. Following the Web Content Accessibility Guidelines (WCAG) is essential.
Semantic HTML
Using semantic HTML elements improves the accessibility and readability of web pages.
Example:
<nav>
<ul>
<li><a href="#home">Home</a></li>
<li><a href="#about">About</a></li>
</ul>
</nav>
ARIA Attributes
Accessible Rich Internet Applications (ARIA) attributes provide additional information to assistive technologies, enhancing accessibility.
Example:
<button aria-label="Close Menu">X</button>
Keyboard Navigation
Ensure that all interactive elements are accessible via keyboard navigation. This can be tested using the tab key to navigate through the webpage.
Optimizing front-end code and ensuring accessibility are fundamental practices in front-end development. These strategies lead to faster loading times, improved performance, and a more inclusive user experience, aligning with best practices and modern web standards.
The Future of Front-End Development
Front-end development is evolving rapidly, driven by innovative trends and technologies that shape modern web experiences. Key trends such as Progressive Web Apps (PWAs) and advanced CSS grid layouts are redefining the field.
Emerging Trends and Technologies
Progressive Web Apps (PWAs) combine the best of web and mobile apps, providing offline access, fast load times, and a native-like user experience. Leveraging Service Workers and Web App Manifests, PWAs are becoming a standard for delivering superior user experiences across devices.
Example of a simple Service Worker registration:
if ('serviceWorker' in navigator) {
navigator.serviceWorker.register('/sw.js').then(function(registration) {
console.log('Service Worker registered with scope:', registration.scope);
}).catch(function(error) {
console.log('Service Worker registration failed:', error);
});
}
CSS grid layouts offer powerful, flexible ways to create responsive and intricate web designs. The grid system provides precise control over layout structure, enabling developers to build complex, adaptable designs with ease.
Employment Opportunities and Salary Prospects
The demand for skilled front-end developers is robust, reflecting the continuous growth of the tech industry. Career paths in front-end development can lead to roles such as UI/UX Designer, Front-End Engineer, and Full-Stack Developer. According to industry reports, salaries for front-end developers range from $70,000 to $120,000 annually, with variations based on experience, location, and expertise in newer web development trends.
The future of front-end development is bright, characterized by technological advancements and promising career opportunities. Staying abreast of emerging trends like PWAs and CSS grid layouts will be crucial for developers aiming to excel in this dynamic field.
Conclusion
In the world of front-end development, staying abreast of the latest trends and technologies is crucial. Embrace the evolving digital façade by continuously updating your skills and knowledge. By doing so, you'll be well-equipped to create cutting-edge, responsive web experiences that meet modern user expectations and industry standards. Engaging with emerging technologies like Progressive Web Apps and advanced CSS techniques will ensure you remain at the forefront of front-end development.
Comments